Last updated on April 11, 2024
Up to previous entry, we’ve successfully connected to DZone RSS Feed and parsed its XML. Given we’re working with mobile devices that could be disconnected from the internet, we want to persist the parsed RSS XML. This is helpful as it allows our app to display the most recently downloaded content when there’s no internet connectivity.
Persisting RSS Content
Following prior tutorial, let’s create a group call “Util” and associate it to a specific file system folder “Util”. Then, add a new file called JSTRRSSUtil, a subclass of NSObject, to this group. Let’s expose a convenience method in our header that will retrieve the root document folder where we can save our parsed RSS content.
#import <Foundation/Foundation.h>
@interface JSTRRSSUtil : NSObject
+ (NSString *) getDocumentRoot;
@end
In Objective-C, character “+” at the start of method declaration indicates this method is a class method. Now let’s implement our class method
#import "JSTRRSSUtil.h"
@implementation JSTRRSSUtil
+ (NSString *) getDocumentRoot
{
// search for available path and return first
NSArray *paths = NSSearchPathForDirectoriesInDomains(NSDocumentDirectory, NSUserDomainMask, YES);
return [paths objectAtIndex:0];
}
@end
Now that we can retrieve our document path, let’s go back to JSTRRSSReader.m and save our content to file. Append the last 2 lines from the connectionDidFinishLoading method.
- (void)connectionDidFinishLoading:(NSURLConnection *)connection
{
NSXMLParser *parser = [[NSXMLParser alloc] initWithData:_receivedData];
JSTRRSSParser *rssParser = [[JSTRRSSParser alloc] init];
parser.delegate = rssParser;
[parser parse];
NSMutableArray *articleList = [rssParser getParsedList];
NSLog(@"%@", articleList);
NSString *fileName = [NSString stringWithFormat:@"%@/popular.rss", [JSTRRSSUtil getDocumentRoot]];
[articleList writeToFile:fileName atomically:YES];
}
In the last two lines of our method, we first construct the fully qualifying file name with the help of JSTRRSSUtil convenience method (Note, you need to import “JSTRRSSUtil.h” for this call to work) and call the writeToFile:atomically method. This will write the content of our array containing a dictionary, each representing a single article.
Run and Verify
Once you run your latest changes, if all works out, your simulator should have written the content of RSS to disk. As the simulator uses your local disk, you can easily verify this by navigating to ~/Library/Application Support/iPhone Simulator/<<simulator version>>/Applications/<<auto generated GUID>>/Documents. In documents Folder, you’ll see our popular.rss file. The content of this file too will be in JSON format and will be safe to open.
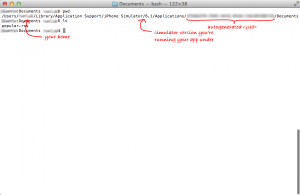